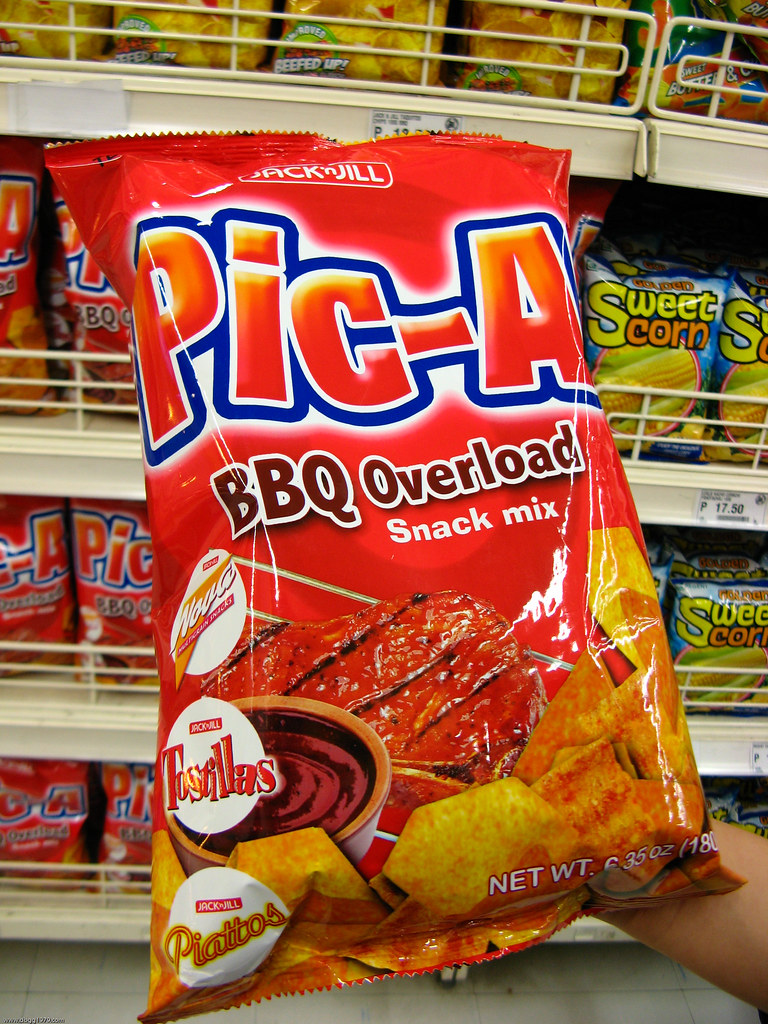
External CTs(1) For motor overload protection applications above 100 A in current sensing capability, the E100 relay offers functionality with external CT configurations up to 800 A maximum capacity (Cat. After 8 months of hard work! The episode is done! Hopefully you guys enjoy! This took a long while!:D-. Overload v1.1.0. 1.1.0; 4f2b7aa; Compare. Choose a tag to compare. Search for a tag. Adriengivry released this Sep 19, 2019 96 commits to master since this release.
Provides a macro to simplify operator overloading.
To use, include the following:
Suppose we have the following struct
definition:
We can overload the addition of Val
s like so:
The macro call above generates the following code:
We are now able to add Val
s:
If we also wanted to overload addition for the borrowed type &Val
we could write: Super vectorizer 2 0 0 download free.
We might also want to overload addition between the owned and borrowed types:
Let's see how we can write these combinations more concisely.
Overlord 1080 X 1080
We can include a ?
in front of a type to indicate that it should stand in for both the owned and borrowed type.
To overload addition for all four combinations between Val
and &Val
we can therefore simply include a ?
in front of both types:
The macro call above generates the following code:
Overlord 1080
We are now able to add Val
s and &Val
s in any combination:
The general syntax to overload a binary operator between types and
is:
Inside the body you can use and
freely to perform any computation.
The last line of the body needs to be an expression (i.e. no ;
at the end of the line) of type .
Operator | Example | Trait |
---|---|---|
+ | overload!((a: A) + (b: B) -> C { /*..*/ ); | Add |
- | overload!((a: A) - (b: B) -> C { /*..*/ ); | Sub |
* | overload!((a: A) * (b: B) -> C { /*..*/ ); | Mul |
/ | overload!((a: A) / (b: B) -> C { /*..*/ ); | Div |
% | overload!((a: A) % (b: B) -> C { /*..*/ ); | Rem |
& | overload!((a: A) & (b: B) -> C { /*..*/ ); | BitAnd |
| | overload!((a: A) | (b: B) -> C { /*..*/ ); | BitOr |
^ | overload!((a: A) ^ (b: B) -> C { /*..*/ ); | BitXor |
<< | overload!((a: A) << (b: B) -> C { /*..*/ ); | Shl |
>> | overload!((a: A) >> (b: B) -> C { /*..*/ ); | Shr |
The general syntax to overload an assignment operator between types and
is:
Inside the body you can use and
freely to perform any computation and mutate
as desired.
Operator | Example | Trait |
---|---|---|
+= | overload!((a: &mut A) += (b: B) { /*..*/ ); | AddAssign |
-= | overload!((a: &mut A) -= (b: B) { /*..*/ ); | SubAssign |
*= | overload!((a: &mut A) *= (b: B) { /*..*/ ); | MulAssign |
/= | overload!((a: &mut A) /= (b: B) { /*..*/ ); | DivAssign |
%= | overload!((a: &mut A) %= (b: B) { /*..*/ ); | RemAssign |
&= | overload!((a: &mut A) &= (b: B) { /*..*/ ); | BitAndAssign |
|= | overload!((a: &mut A) |= (b: B) { /*..*/ ); | BitOrAssign |
^= | overload!((a: &mut A) ^= (b: B) { /*..*/ ); | BitXorAssign |
<<= | overload!((a: &mut A) <<= (b: B) { /*..*/ ); | ShlAssign |
>>= | overload!((a: &mut A) >>= (b: B) { /*..*/ ); | ShrAssign |
Apple remote desktop 3 9 32. The general syntax to overload a unary operator for type is:
Inside the body you can use freely to perform any computation.
The last line of the body needs to be an expression (i.e. no ;
at the end of the line) of type .
Operator | Example | Trait |
---|---|---|
- | overload!(- (a: A) -> B { /*..*/ ); | Neg |
! | overload!(! (a: A) -> B { /*..*/ ); | Not |
Remember that you can only overload operators between one or more types if at least one of the types is defined in the current crate.
Macros
overload | Overloads an operator. See the module level documentation for more information. |